Lab 4: A first look at Big-O notation; Sorting Analysis
- Task 1: Practice algorithm analysis
- Task 2: Review and Analyze Bubblesort
- Task 3: Practice with sorting
- Task 4: Practice merge sort
- Task 5: Work with a linked list of characters
- Task 6: Understand assignments involving references
- Post Lab Tasks (on Linked Lists)
- Task 7: Trace an iterative linked-list method
- Task 4: Convert an iterative method to a recursive one
Task 1: Practice algorithm analysis
Consider the following code fragment:
for (int i = 0; i < n; i++) { for (int j = 0; j < 2*n; j++) { methodA(); for (int k = 0; k < n + 1; k++) { methodB(); } } for (int j = 0; j < i; j++) { methodC(); } if (i % 2 == 0) { methodD(); } }
For each of the following, find the function that expresses the
number of times each part of the code is executed for a given
value of n
, and then determine the corresponding big-O expression.
- the number of times
methodA()
is called - the number of times
methodB()
is called - the number of times
methodC()
is called - the number of times
methodD()
is called
Task 2: Review and Analyze Bubblesort
In lecture, you have begun to learn about some of the many algorithms that are available for sorting arrays of values.
Now let’s take a closer look at Bubble sort. On each pass, the bubblesort algorithm proceeds from left to right, swapping adjacent elements if they are out of order. As a result, larger elements “bubble up” to the end of the array.
For example, consider the following array:
28 | 24 | 27 | 18 |
First pass Bubble sort compares elements 0 and 1 (the 28 and 24), and because they are out of order, it swaps them:
24 | 28 | 27 | 18 |
It then compares elements 1 and 2 (the 28 in its new position and 27), and because they are out of order, it swaps them:
24 | 27 | 28 | 18 |
Finally, it compares elements 2 and 3 (the 28 in its new position and 18), and because they are out of order, it swaps them:
24 | 27 | 18 | 28 |
Note that the largest element (the 28) has bubbled up to its final position, so we don’t need to consider it on the next pass.
Second pass Bubble sort compares the elements 0 and 1 (the 24 and 27), and because they are in order, it leaves them alone:
24 | 27 | 18 | 28 |
It then compares elements 1 and 2 (the 27 and 18), and because they are out of order, it swaps them:
24 | 18 | 27 | 28 |
And the second pass ends there.
Note that the second largest element (the 27) has bubbled up to its final position, so we don’t need to consider it on the next pass.
Third pass Bubble sort compares elements 0 and 1 (the 24 and 18), and because they are out of order, it swaps them:
18 | 24 | 27 | 28 |
And the third pass ends there.
Note that the third largest element (the 27) has bubbled up to its final position, so we don’t need to consider it on the next pass.
And because there is only one remaining element that hasn’t been bubbled up, the algorithm ends, and the array is sorted.
-
Trace bubble sort on the following array:
7 39 20 11 16 5 Show what the array looks like after each swap that occurs.
-
Here is the implementation of bubble sort from our
Sort
class:public static void bubbleSort(int[] arr) { for (int i = arr.length - 1; i > 0; i--) { for (int j = 0; j < i; j++) { if (arr[j] > arr[j+1]) { // how many comparisons? swap(arr, j, j+1); } } } }
Note that:
-
The inner loop performs a single pass.
-
The outer loop governs the number of passes, and the ending point of each pass.
Determine a formula for the number of comparisons of array elements that bubble sort performs when operating on an array of length
n
.To do so, it can help to begin by filling in a table like this one:
pass # # of comparisons ------ ---------------- 1 n - 1
-
Fill in the next few rows of this table, and see if you notice a pattern.
-
How can we take the pattern revealed by the table and use it to derive an exact formula for the number of comparisons?
-
Given that formula, what is the big-O expression for the number of comparisons performed by bubble sort?
-
The number of moves performed by bubble sort depends on the contents of the array. How many are performed as a function of n:
- in the best case?
- in the worst case?
- in the average case?
-
What is the big-O expression for the overall running time of bubble sort?
-
What changes to the algorithm would be required to optimize it such that the algorithm can run
O(n)
in the best case?
-
Task 3: Practice with sorting
In this task, we will practice some of the algorithms from our Sort class.
Consider the following array:
7 | 39 | 20 | 11 | 16 | 5 |
-
Trace insertion sort on the above array.
Show what the array looks like after each iteration of the outer loop of the algorithm (i.e., after each element is considered for insertion and either left alone or inserted). -
Recall that quicksort begins by partitioning the array with respect to a pivot value. Trace through the initial partitioning of the array shown above.
-
Now complete the trace of quicksort on this array by filling in the diagram below.
Task 4: Practice merge sort
Like quicksort, merge sort uses a recursive, divide-and-conquer approach. However, whereas quicksort does all of its work during the divide stage (before making the recursive calls), merge sort instead does all of its work after a given set of recursive calls have returned, as it merges sorted subarrays.
-
Trace through mergesort on the following array:
----------------------------------------- | 7 | 39 | 20 | 11 | 16 | 5 | 9 | 28 | ----------------------------------------- split / \ --------------------- --------------------- | | | | | | | | | | --------------------- --------------------- split split / \ / \ ----------- ----------- ----------- ----------- | | | | | | | | | | | | ----------- ----------- ----------- ----------- split split split split / \ / \ / \ / \ ------ ------ ------ ------ ------ ------ ------ ------ | | | | | | | | | | | | | | | | ------ ------ ------ ------ ------ ------ ------ ------ \ / \ / \ / \ / merge merge merge merge ----------- ----------- ----------- ----------- | | | | | | | | | | | | ----------- ----------- ----------- ----------- \ / \ / merge merge --------------------- --------------------- | | | | | | | | | | --------------------- --------------------- \ / ----------------------------------------- | | | | | | | | | -----------------------------------------
-
What major advantage does merge sort have over quicksort with respect to time complexity?
-
What major disadvantage does merge sort have compared to quicksort with respect to space complexity?
Task 5: Work with a linked list of characters
In lecture, we’ve been considering linked lists of characters, where each node in the linked list is an instance of the following class:
public class StringNode { private char ch; private StringNode next; ... }
For example, we considered the following linked list, which represents the
string "dog"
:
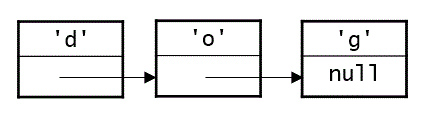
Note that each node in the linked list has two fields:
-
the top field (the field
ch
), which stores a single character -
the bottom field (the field
next
), which is a reference of typeStringNode
. Thesenext
fields link the nodes together. They either hold a reference to the next node in the linked list, or (in the case of the last node) they have a value ofnull
to indicate that there are no subsequent nodes.
Your tasks
-
Consider the following diagram, which is a visualization of a heap memory region in which a number of
StringNode
objects have been created. Each node includes the values of itsch
andnext
fields, as well its starting position in memory.0xc000 0xbe00 0x3004 +--------+ +--------+ +--------+ ch | 'a' | ch | 'b' | ch | 'c' | +--------+ +--------+ +--------+ next | 0xbe00 | next | 0x3004 | next | 0xbb00 | +--------+ +--------+ +--------+ 0xa004 0xff00 0xbb00 +--------+ +--------+ +--------+ ch | 'd' | ch | 'e' | ch | 'f' | +--------+ +--------+ +--------+ next | 0xff00 | next | null | next | 0xa004 | +--------+ +--------+ +--------+
Important notes:
-
Instead of using arrows to represent each node’s
next
field, we have written the actual value of each reference (ornull
, in the case of no reference). We have written these reference values as hexadecimal (base 16) numbers that begin with0x
. -
The order of the nodes in the diagram does not necessarily correspond to the order of the nodes in the linked list. This is also true when you create a linked list in your program. The nodes are not necessarily next to each other in memory. They can be located at arbitrary locations on the heap. That is why each node must maintain a reference to the next node!
-
-
Convert the diagram above to one that looks like the diagrams from lecture, in which:
-
the nodes are shown in a single chain stretching from the first node to the last node in the linked list
-
references are drawn using arrows
Your new diagram should still include the memory address of the start of each node.
Note: The node containing the letter
'a'
is the first node in the linked list. To determine the order of the remaining nodes, follow thenext
references! -
-
Suppose that we have two variables in the
main
method of ourStringNode
class:-
one called
n
, which holds a reference to the node at address0xbe00
-
one called
m
, which holds a reference to the node at address0xbb00
Add the variables
n
andm
to the diagram you created for question 2. -
-
In the rest of this task, we will determine both the address and the value of several fields from our diagram.
To do so, we will make the following assumptions:
-
the memory address of the
ch
field of aStringNode
is the same as the address of theStringNode
itself -
the memory address of the
next
field of aStringNode
is 2 more than the address of theStringNode
itself.
Complete the table shown below, filling in the address and value of the field specified by each expression from the left-hand column. We have done the first two expressions for you.
expression address value ---------- ------- ----- m.ch 0xbb00 'f' m.next 0xbb02 0xa004 (reference to the 'd' node) m.next.next n.next n.next.ch n.next.next.next
-
Task 6: Understand assignments involving references
To determine the effect of an assignment statement involving references, it can help to use the following procedure:
-
Identify the two boxes – i.e., the variables/fields specified by the expressions on both sides of the assignment operator.
-
Determine the value in the box specified by the expression on the right-hand side.
-
Copy that value into the box specified by the expression on the left-hand side.
Beginning with our diagram from Task 1:
-
Draw the diagram that results from the following assignment:
n = m.next;
-
Now modify the drawing to show the result of the following:
m.next = m.next.next
Post Lab Tasks (on Linked Lists)
Task 7: Trace an iterative linked-list method
The StringNode
class includes a method
called convert
that uses iteration to gradually convert a Java String
object to a linked-list string:
public static StringNode convert(String s) { if (s.length() == 0) { return null; } // Create the head node StringNode firstNode = new StringNode(s.charAt(0), null); // These two variables are needed to traverse down // the list as each "new" node created is linked to the // "previous" node. StringNode prevNode = firstNode; StringNode nextNode; // Loop through the remaining characters of the string, // create a new node for each character and, // link it to the previous node. for (int i = 1; i < s.length(); i++) { // Create the next node nextNode = new StringNode(s.charAt(i), null); // Link it to the previous node prevNode.next = nextNode; // Update the previous node prevNode = nextNode; } // Return the first node - head of the list return firstNode; }
Note that the method uses several variables of type StringNode
:
-
one called
firstNode
, which holds a reference to the node containing the first character; we need this variable so that the method can return a reference to the first node after the entire linked list has been created -
one called
nextNode
, which is used to hold a reference to each new node that is created (except the first node) -
one called
prevNode
, which starts out pointing to the first node, and which stays one node behindnextNode
in the linked list
Let’s trace through the execution of the following call of this method:
StringNode s1 = StringNode.convert("node");
Task 4: Convert an iterative method to a recursive one
Now rewrite the convert()
method. Remove the existing iterative
implementation of the method, and replace it with one that uses
recursion instead.
Your new method should be much simpler! It should take advantage of
our usual recursive approach to processing strings – both Java String
objects, and strings that are represented as linked lists:
-
base case: if the string is empty, handle it directly
-
recursive case: take care of the first character, and make a recursive call to handle the rest of the string.
To test your recursive version of the method, add statements like
the following to your testDriver main
method:
StringNode s = StringNode.convert("node"); System.out.println(s);
(Note: We are able to see the string when we print ou the node because our
StringNode
class includes a toString()
method.)